I’ve been reviewing automated testing tools I previously used, to discover what I can use for Hugo. Automated testing fits in well with Hugo along with automation of the build and site deployment. Using Selenium IDE (a browser extension / screen recorder) you can first record the test case and then when development is finished, you just run the automated test. If the test automation completes without errors - then you can say thanks for a job well done.
One of the great things with Hugo is the Auto Reload function when the server is running locally - if anything is broken, the server generates an error for you to fix. But what about checks before the site is published? What do you click around and check? Wouldn’t it be great if you could automate that and reduce your manual efforts
Say hello to Selenium.
In this guide you will …
- Install Selenium, web drivers and relevant Python test packages
- Write your first Selenium Python test case
- Run pytest and with confidence complete an automated tests
Just also recognise - if Python isn’t your thing Selenium also does Java, Ruby and other languages. I chose Python because I found it easy to read.
Getting Started - Tools and Packages
If you have missed my articles to get started on testing … that’s ok - But make sure you have Homebrew on your machine.
From the terminal …
brew install Python
If you have a Mac you may now have Python 2 and Python 3 on your machine, that’s ok. From now on we will always use Python3
Then … let’s add the web drivers - these control the browsers:
|
|
You need both browsers on your machine
Install Selenium and Python Packages
|
|
Note
- Pip3 is the Python3 Package Manager
- Pytest is a test framework for Python
- Pytest-html is plugin that generates an HTML report for the test results
Basic Python Selenium Test with Pytest
This is a very basic test that
- opens Chrome
- saves the Title of the Page
- takes a screenshot
- does the same in Firefox
So…
- Open your Code / Development Environment
- Copy/paste the code below
- Change the highlighted lines to your website and then
- Save the file .. ideally to /tests/test_selenium_basic_.py
|
|
Note
- you need both browsers installed or this will fail
Run your First Pytest Selenium Test
Go to terminal
|
|
Pytest runs all .py files in the directory … or you can be specific
pytest test_selenium_basic.py --html=report.html
All going well you’ll first see Firefox open and then Chrome. After which, your terminal will show:
|
|
And if you view the test report …
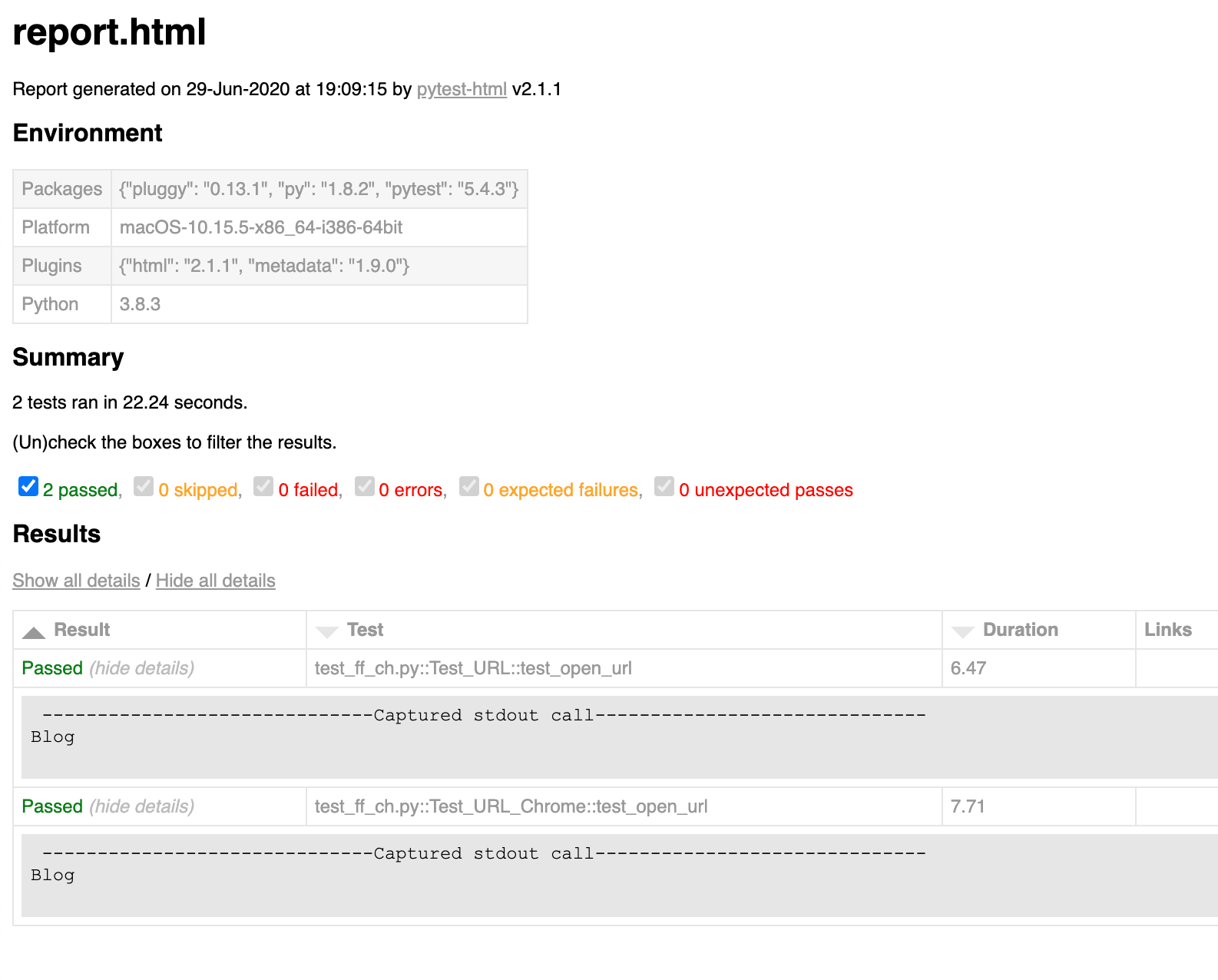
Use Selenium IDE to record tests then export to Python
If you’re not much of a coder … and want to explore more options of testing - you can record your tests in Selenium and then save them as Python tests scripts … which is awesome
Test and Go
So now …
- Save the file - for consistency, test cases are always saved under
/tests/
- Commit your files to Git
- Run the test
- Relax knowing you’re site is in good hands
Linkage
Tags:Contact me today to find out how I can help you.